
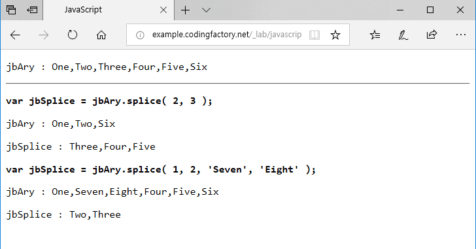
shift will do the same, but return the element itself, not an array. If you want to insert another array into an array without creating a new one, the easiest way is to use either push or unshift with apply. If you do not specify any elements, splice() will only remove elements from the array. splice (0, 1) will remove the first element from myarray and return a new array containing that element. const arrayEmpty new Array(2) console.log(arrayEmpty.length) // 2 console.log(arrayEmpty0) // undefined actually, it is an empty slot console.log(0 in. An array is created with its length property set to that number, and the array elements are empty slots. The elements to add to the array, beginning from start. Arrays can be created using a constructor with a single number parameter. In this case, you should specify at least one new element (see below). If deleteCount is 0 or negative, no elements are removed. () is used to slice an array from the start point to the end point, excluding the end. However, if you wish to pass any itemN parameter, you should pass Infinity as deleteCount to delete all elements after start, because an explicit undefined gets converted to 0. Please note that, if multiple elements are passed as parameters, they're inserted in chunk at the beginning of the object, in the exact same order they were. () has similar behavior to unshift (), but applied to the end of an array. If deleteCount is omitted, or if its value is greater than or equal to the number of elements after the position specified by start, then all the elements from start to the end of the array will be deleted. The unshift () method inserts the given values to the beginning of an array-like object. This is different from passing undefined, which is converted to 0.Īn integer indicating the number of elements in the array to remove from start. If start is omitted (and splice() is called with no arguments), nothing is deleted.
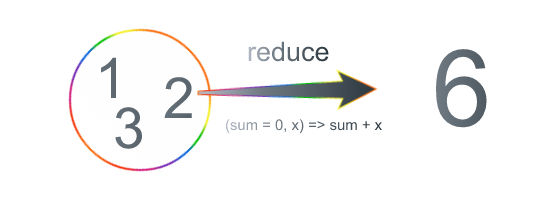
Negative index counts back from the end of the array - if start = array.length, no element will be deleted, but the method will behave as an adding function, adding as many elements as provided.This parameter is the index from which the modification of the array starts (with the origin at 0). If the sliced portion is sparse, the returned array is sparse as well. The slice () method preserves empty slots. Zero-based index at which to start changing the array, converted to an integer. Syntax: Array.splice ( index, removecount, itemlist ) Parameter: This method accepts many parameters some of which are described below: index: It is a required parameter. It does not alter this but instead returns a shallow copy that contains some of the same elements as the ones from the original array. This method modifies the original array and returns the removed elements as a new array. It lets you change the content of your array by removing or replacing existing elements with new ones. Object.prototype._lookupSetter_() Deprecated The splice () method is a built-in method for JavaScript Array objects.Object.prototype._lookupGetter_() Deprecated.Object.prototype._defineSetter_() Deprecated.
